Figure 5 script
Contents
Figure 5 script¶
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
import seaborn as sns
import matplotlib as mpl
# font and linewidth settings
font = {'size' : 20}
mpl.rc('font', **font)
mpl.rc('legend', fontsize=16)
axes_settings = {'linewidth' : 2}
mpl.rc('axes', **axes_settings)
Now read the data:
df = pd.read_csv('../results_2022.csv', dtype=str)
df = df.replace('varying: multi-pass', 0) # replace some values in 'Template size (m)' with another flag
for field in ['Pixel spacing (m)',
'Template size (m)',
'SAV-uncertainty-x',
'SAV-uncertainty-y',
'SAV-peak-x',
'SAV-peak-y',
'LSR-uncertainty-nm',
'LSR-uncertainty-sh',
'pt0_vxdiff',
'pt0_vydiff',
'pt1_vxdiff',
'pt1_vydiff',
'pt2_vxdiff',
'pt2_vydiff',
'pt0_vxavgdiff',
'pt0_vyavgdiff',
'pt1_vxavgdiff',
'pt1_vyavgdiff',
'pt2_vxavgdiff',
'pt2_vyavgdiff',
'SAV-outlier-percent',
'Invalid-pixel-percent']:
df[field] = df[field].astype(float)
datestrs = ['LS8-20180304-20180405',
'LS8-20180802-20180818',
'Sen2-20180304-20180314',
'Sen2-20180508-20180627']
# df
Create additional column fields for grouping data:
df['large_vxdiff'] = np.abs(df['pt0_vxdiff']) > df['SAV-uncertainty-x']
df['large_vydiff'] = np.abs(df['pt0_vydiff']) > df['SAV-uncertainty-y']
df['large_vxavgdiff'] = np.abs(df['pt0_vxavgdiff']) > df['SAV-uncertainty-x']
df['large_vyavgdiff'] = np.abs(df['pt0_vyavgdiff']) > df['SAV-uncertainty-y']
df['Invalid+Incorrect'] = df['Invalid-pixel-percent'] + df['SAV-outlier-percent'] * (1 - df['Invalid-pixel-percent'] / 100)
df['large_vxdiff'] = df['large_vxdiff'].astype(str)
df['large_vxavgdiff'] = df['large_vxavgdiff'].astype(str)
fig, axs = plt.subplots(1, 2, figsize=(16, 7), constrained_layout=True)
axs[0].axhline(y=0.004, linestyle='--', color='gray')
sns.scatterplot(data=df, x='SAV-uncertainty-x', y='LSR-uncertainty-sh', hue='Invalid+Incorrect', palette="mako_r", ax=axs[0], s=60)
axs[0].set_ylim(0, 0.06)
axs[0].set_xlim(0, 0.8)
sns.stripplot(data=df, x="LSR-uncertainty-sh", y="large_vxdiff", s=8, alpha=0.5,
ax=axs[1])
kawgs2 = {'linewidth': 3, }
tmp = df[df['large_vxdiff'] == 'False']
tmp_mean = tmp['LSR-uncertainty-sh'].median()
axs[1].plot([tmp_mean, tmp_mean], [-0.3, 0.3], **kawgs2)
tmp = df[df['large_vxdiff'] == 'True']
tmp_mean = tmp['LSR-uncertainty-sh'].median()
axs[1].plot([tmp_mean, tmp_mean], [0.7, 1.3], **kawgs2)
axs[1].set_xlim(0, 0.025)
axs[0].text(-0.16, 1, "$\mathbf{a}$", transform=axs[0].transAxes)
axs[1].text(-0.16, 1, "$\mathbf{b}$", transform=axs[1].transAxes)
axs[0].set_xlabel('$\delta_u$ (m day$^{-1}$)')
axs[0].set_ylabel("$\delta_{x'y'}$ (day$^{-1}$)")
axs[0].get_legend().set_title("Invalid & incorrect \n matches (%)")
axs[1].set_xlabel("$\delta_{x'y'}$ (day$^{-1}$)")
axs[1].set_ylabel("Oberved flow speed deviation from \n ground truth (GNSS) larger than $\delta_u$?")
# save figure
fig.patch.set_facecolor('xkcd:white')
fig.savefig('Fig5.png', dpi=200)
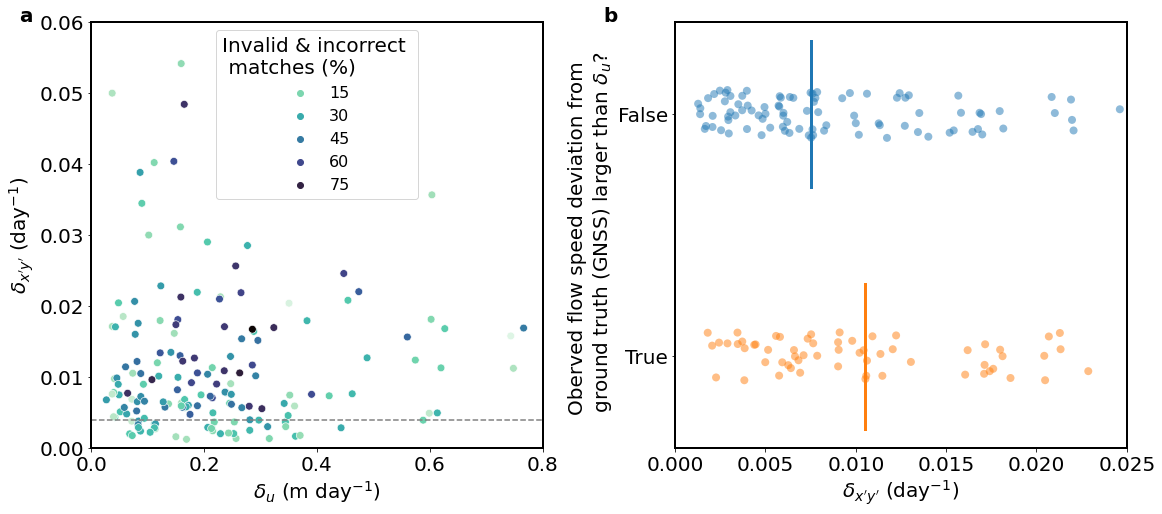
Additional notes¶
If we replace
large_vxdiff
withlarge_vxavgdiff
(which is the status averaged from the nearest 3x3 array), the results won’t change much.
kawgs2 = {'linewidth': 3, }
fig, ax = plt.subplots(1, 1, figsize=(7, 7))
sns.stripplot(data=df, x="LSR-uncertainty-sh", y="large_vxavgdiff", s=8, alpha=0.5, ax=ax)
tmp = df[df['large_vxavgdiff'] == 'False']
tmp_mean = tmp['LSR-uncertainty-sh'].median()
ax.plot([tmp_mean, tmp_mean], [-0.3, 0.3], **kawgs2)
tmp = df[df['large_vxavgdiff'] == 'True']
tmp_mean = tmp['LSR-uncertainty-sh'].median()
ax.plot([tmp_mean, tmp_mean], [0.7, 1.3], **kawgs2)
ax.set_xlim(0, 0.025);

Here shows how the static-area correct-match uncertainty (\(\delta_x\), gray bar) compares to the ground truth deviation at the GNSS station (pt0) for each test pair.
fig, ax = plt.subplots(1, 1, figsize=(19, 7))
plt.bar(df.index, df['SAV-uncertainty-x'], color='gray')
plt.bar(df.index, -df['SAV-uncertainty-x'], color='gray')
plt.plot(df.index, df['pt0_vxdiff'], '.', color='xkcd:orange', markersize=10)
plt.xlim(-1, 172)
plt.xlabel('Test #')
plt.ylabel('Orange: sampled velocity from each test - GNSS (m/d) \n gray: 2-sigma uncertainty by the static area velocities');
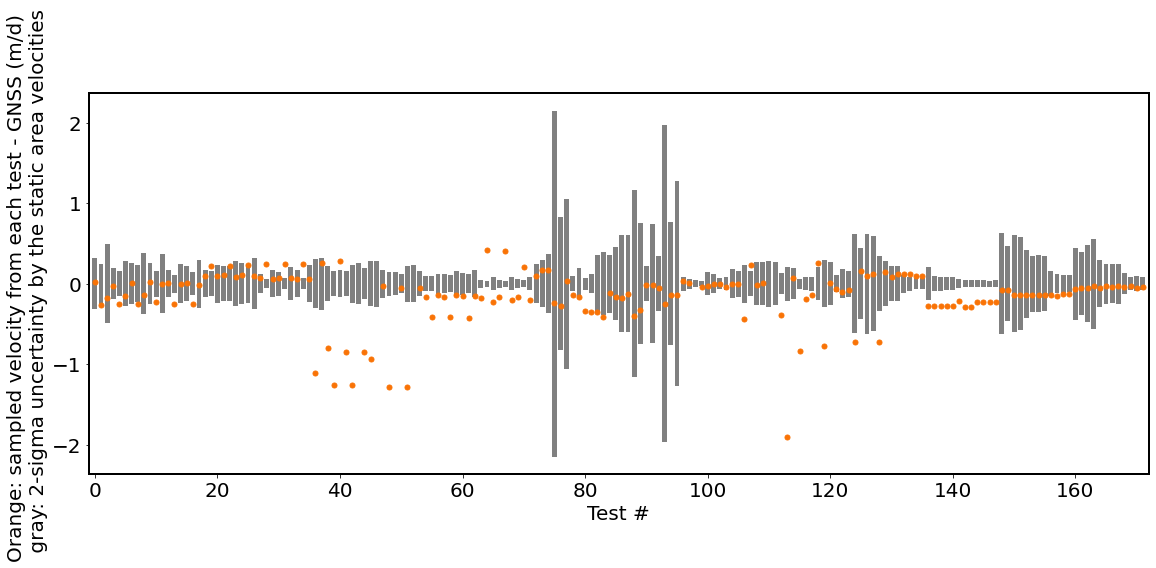